Angular Material Tutorial - 29 - Data table Filtering
Summary
TLDRThis video script offers a concise guide on implementing filtering in Angular Material tables. It outlines a three-step process: creating a data source with the MatTableDataSource class, adding an input field with MatInput for user text input, and defining an 'applyFilter' method to update the data source's filter property. The script demonstrates how assigning a filter value triggers the data source to display only rows containing the filter text, enhancing the user experience by making data navigation straightforward and efficient.
Takeaways
- ð Angular Material simplifies data table functionalities like filtering, sorting, and pagination.
- ð To implement filtering, first create a data source using `MatTableDataSource`.
- ð Instantiate a `MatTableDataSource` and pass in the data as an argument.
- ð Add an input field with `mat-form-field` for users to enter filter text.
- ð¡ Utilize the `keyUp` event to trigger filtering as the user types.
- ð¯ The `applyFilter` method is called on `keyUp`, using the input value.
- ð The `applyFilter` method sets the filter property of the `MatTableDataSource`.
- ðœ The data source automatically filters rows based on the provided filter value.
- ð¢ Filtering can be applied to both text and numeric values in the data table.
- ð Angular Material's `MatTableDataSource` class handles the logic for filtering.
- ð Further videos will cover sorting and pagination functionalities in data tables.
Q & A
What are the main features of Angular Material that the video script discusses?
-The video script discusses the features of filtering, sorting, and pagination in Angular Material. These features simplify the process of managing data in a table format.ã1ã
How does the video script suggest to start implementing filtering in Angular Material?
-The video script suggests that the first step to implement filtering is to create a data source as an instance of the MatTableDataSource class.ã1ã
What is the second step mentioned in the video script for setting up a filter?
-The second step is to create an input field where the user can enter the filter text, using the mat-form-field and mat-input attributes in the HTML.ã1ã
What event should be listened to in order to filter the data table?
-The 'keyup' event should be listened to in order to filter the data table based on the user's input.ã1ã
What method is called when the user types text in the filter input?
-The method called when the user types text in the filter input is 'applyFilter'.ã1ã
How is the 'applyFilter' method defined in the video script?
-The 'applyFilter' method is defined in the component class, and it accepts the filtered text as a string. It then assigns the filter text value to the 'filter' property of the data source.ã1ã
What does the 'MatTableDataSource' class do when a filter value is assigned to its 'filter' property?
-When a filter value is assigned to the 'filter' property of the MatTableDataSource class, it reduces each row to a serialized form and filters out the row if it does not contain the filtered value.ã1ã
How does the filter work in practice according to the video script?
-In practice, the filter works by checking if the data row contains the filtered value. If it does, the row is displayed; if not, it is filtered out. This can be demonstrated by typing letters or numbers into the filter input and seeing the table update accordingly.ã1ã
What will be covered in the subsequent videos following the one described in the script?
-In the subsequent videos, sorting and pagination features in Angular Material's data tables will be covered.ã1ã
How does the video script describe the process of filtering as a whole?
-The video script describes the process of filtering as a three-step process: creating a data source instance, creating an input filter, and assigning the filter value to the data source's filter property.ã1ã
What is the purpose of the 'filterValue.trim().toLowerCase()' in the 'applyFilter' method?
-The 'filterValue.trim().toLowerCase()' in the 'applyFilter' method is used to standardize the filter value by removing any whitespace and converting it to lowercase, ensuring that the filtering is case-insensitive and clean.ã1ã
Outlines
ð Simplifying Filtering, Sorting, and Pagination in Angular Material
This paragraph introduces the ease of implementing filtering, sorting, and pagination features in Angular Material. It outlines a three-step process to achieve filtering in a data table. First, it explains how to create a data source using the MatTableDataSource class from Angular Material. Next, it describes the creation of an input field for user-provided filter text, utilizing the mat-form-field and mat-input attributes. The paragraph then details the implementation of the 'applyFilter' method, which listens for key events and filters the data based on the entered text. The summary emphasizes the functionality of the MatTableDataSource class, which filters data rows based on the presence of the filter string, providing a seamless user experience for data table interactions.
Mindmap
Keywords
ð¡Angular Material
ð¡Filtering
ð¡Sorting
ð¡Pagination
ð¡Data Source
ð¡MatTableDataSource
ð¡mat-form-field
ð¡Keyup Event
ð¡applyFilter Method
ð¡Filter Text
ð¡Data Table
ð¡Serialization
Highlights
Features like filtering, sorting, and pagination have been simplified in Angular Material.
The video demonstrates filtering in Angular Material, with sorting and pagination to be covered in subsequent videos.
Filtering is achieved through three simple steps using Angular Material's mat table data source class.
The first step is to import MatTableDataSource from Angular Material.
Create a data source property as an instance of MatTableDataSource, passing in element data as the argument.
The second step involves creating an input field with mat-form-field and mat-input attributes for the user to enter filter text.
Listen to the 'key' event to filter the data table when the user types in the input field.
The 'applyFilter' method is called on the 'keyup' event, using the filter text accessed by event.target.value.
The final step is to define the 'applyFilter' method that filters the data source by assigning the filter text to the data source's filter property.
The MatTableDataSource class has a filter property that, when assigned a string, filters out rows not containing the filtered value.
Assigning a filter value to the data source's filter property reduces each row to a serialized form and filters out rows that do not contain the value.
The data source will display a row only if it contains the filter value.
The video provides a practical example of filtering elements with the letter 'H' and numeric values '6'.
The video concludes with a preview of the next topic, which is sorting in data tables.
Transcripts
features like filtering sorting and
pagination have been made really simple
in angular material let's take a look at
filtering in this video sorting and
pagination in subsequent videos
filtering can be achieved in three
simple steps the first step is to create
a data source as an instance of the mat
table data source class so let's begin
by importing mat table data source from
angular material next we create a data
source property as an instance of this
imported class so data source is going
to be new mat table data source and then
we pass in element data as the argument
so that is our first step creating the
data source as an instance of the mat
table data source class second step is
to create an input field where the user
can enter the filter text let's add that
code in the HTML mat form field then an
input element we add the mat input
attribute and also a placeholder that
says filter every time the user enters
some text we need to filter the data
table for that we listen to the key of
event
so keep up and on keep up we are going
to call a method called apply filter and
to this method we pass in the filter
text which is accessed using dollar
event dot target dot value that is the
second step creating a filter input the
final step is to define this apply
filter method which actually filters the
data source so back in the component
class apply filter accepts the filtered
text of type string and within the
method all we have to do is assign the
filter text value to the filter property
of the data source so this dot data
source dot filter is equal to filter
value dot trim dot to lowercase now this
kind of seems like magic but let me tell
you how it works remember the math table
data source class that we imported well
that class has a property called filter
when you want to filter out the data all
you have to do is assign a string to
that property when you assign the filter
value the data source will reduce each
row to a serialized form and will filter
out the row if it does not contain that
filtered value or to put it in simpler
terms does the data rope contain the
filter if yes only then display the row
so if you take a look at the browser you
can see that we have a filter input I
type H and you can see it filters the
elements type H E and it filters further
I can also filter on numeric values type
6 and you can see it filters out the
rows that don't contain the number 6 so
three basic steps for filtering creating
the data source as an
instance of math table datasource
creating the input filter and finally on
care of that input element assign the
filter value to the filter property of
the data source alright in the next
video let's take a look at sorting in
data tables
5.0 / 5 (0 votes)
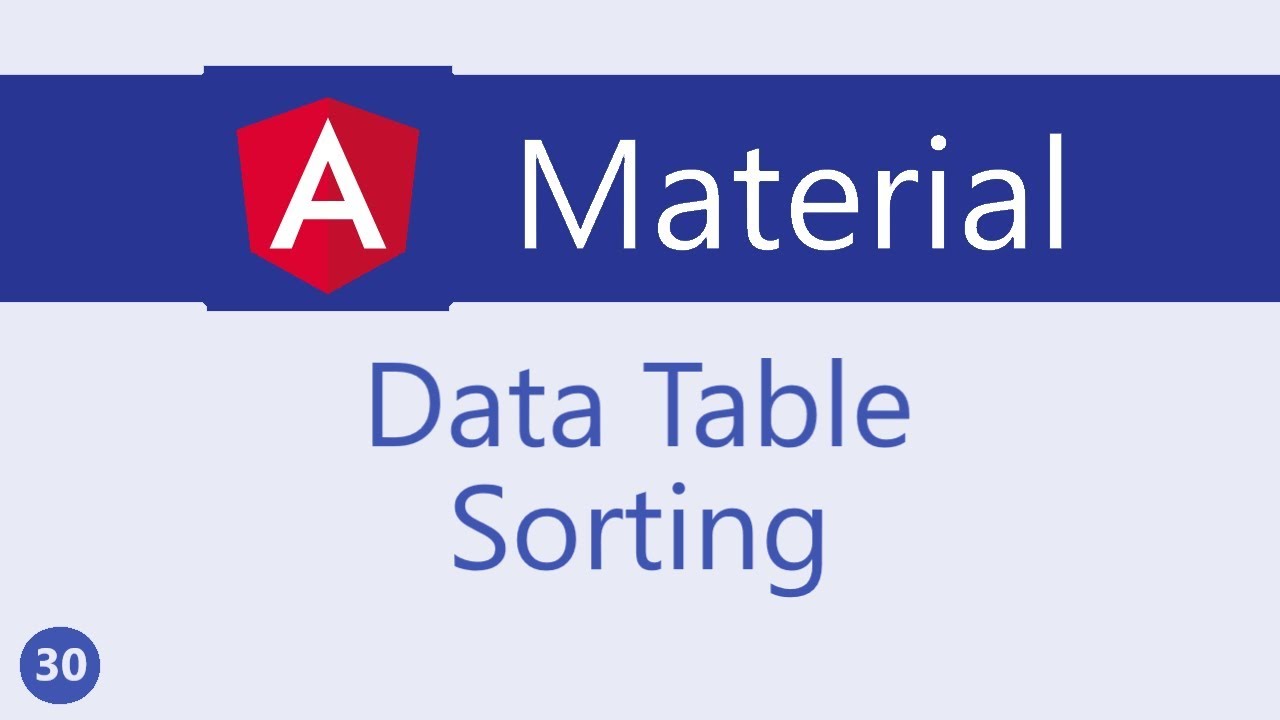
Angular Material Tutorial - 30 - Data table Sorting
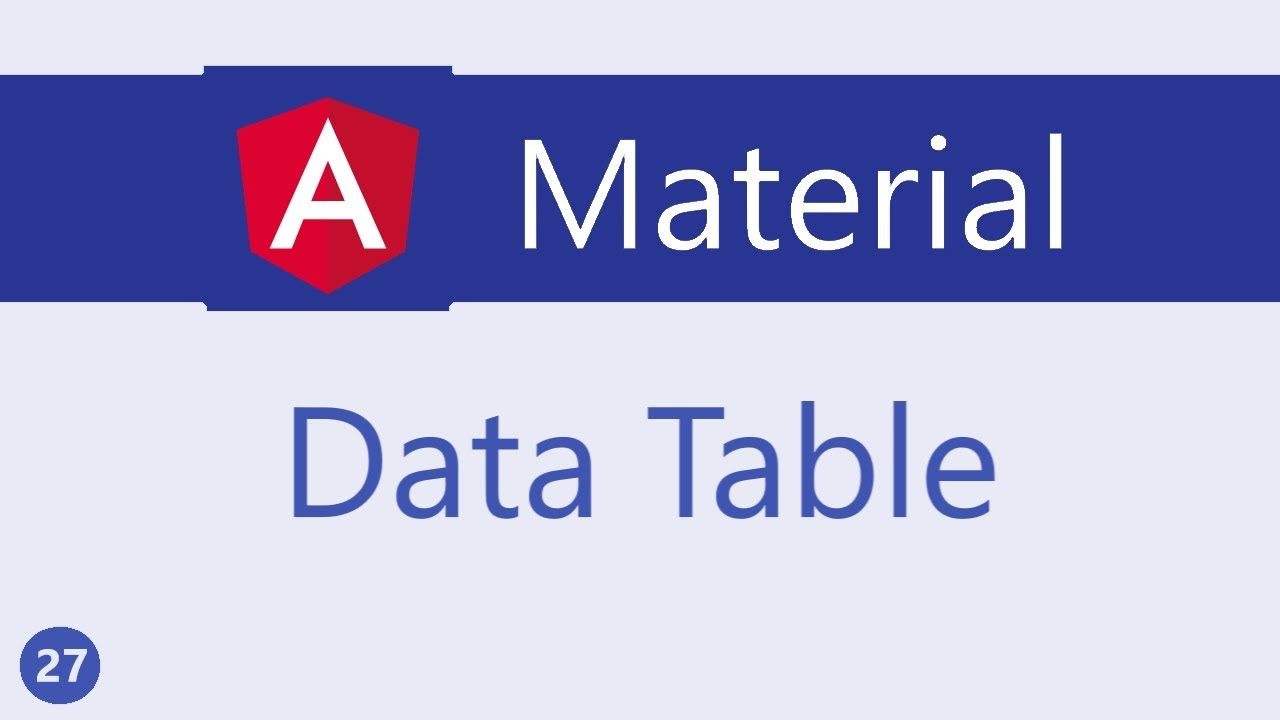
Angular Material Tutorial - 27 - Data table
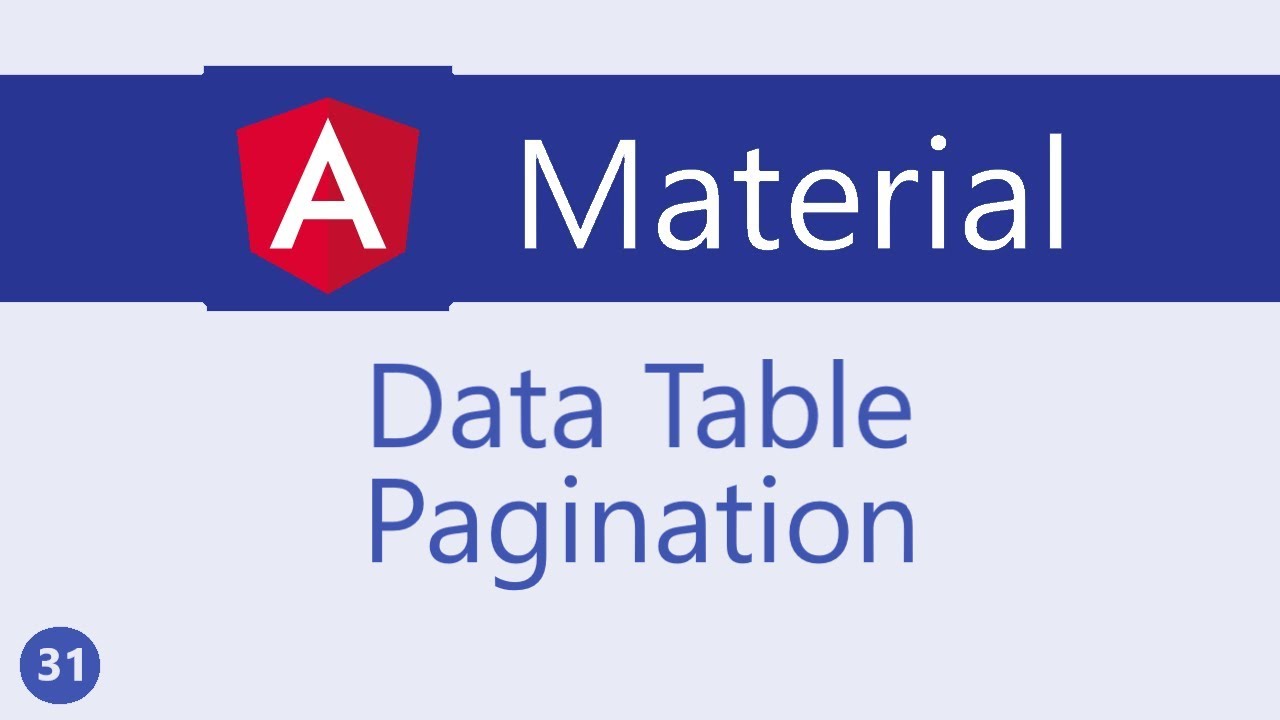
Angular Material Tutorial - 31 - Data table Pagination

Angular Material Tutorial - 19 - Input
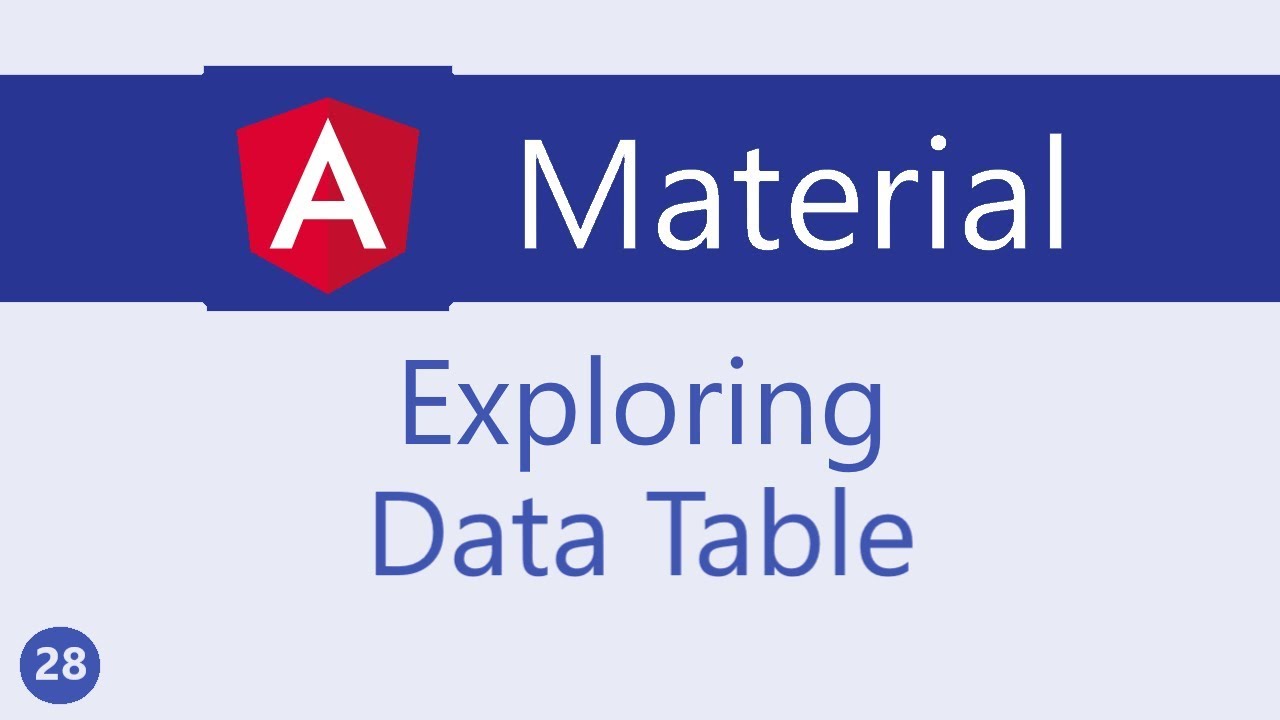
Angular Material Tutorial - 28 - Exploring Data table
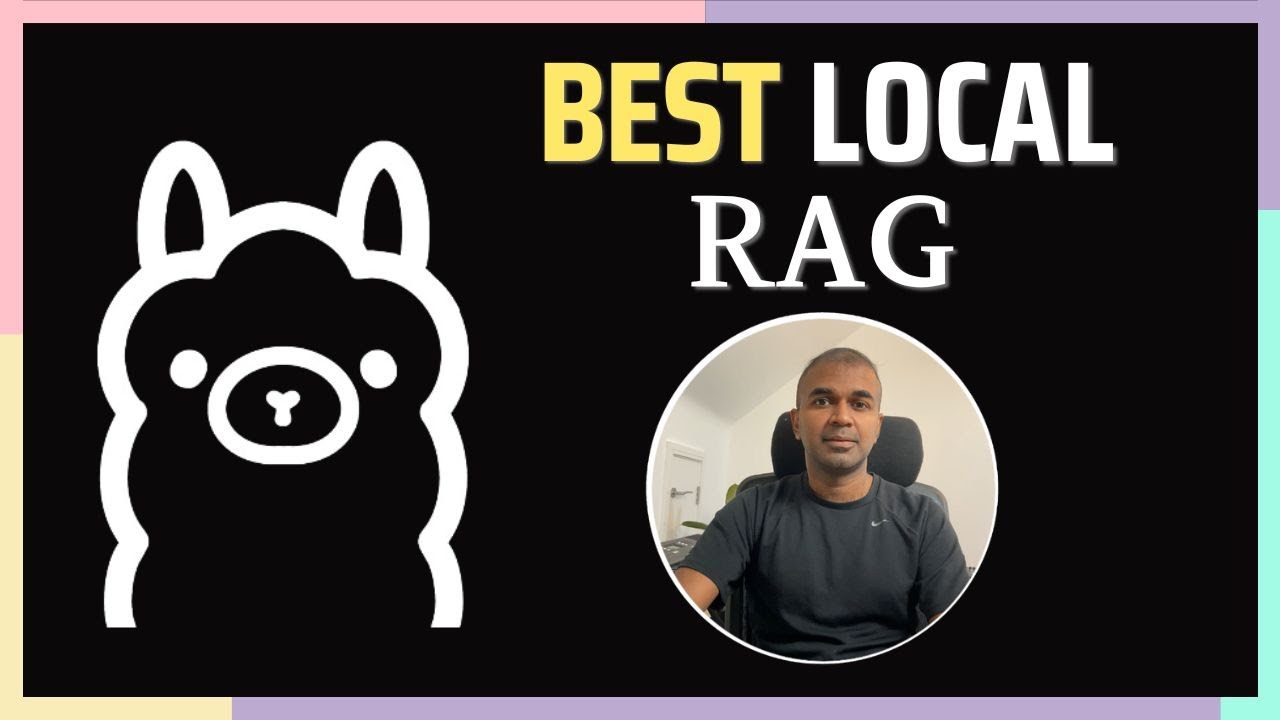
Ollama Embedding: How to Feed Data to AI for Better Response?